Loading different security configurations
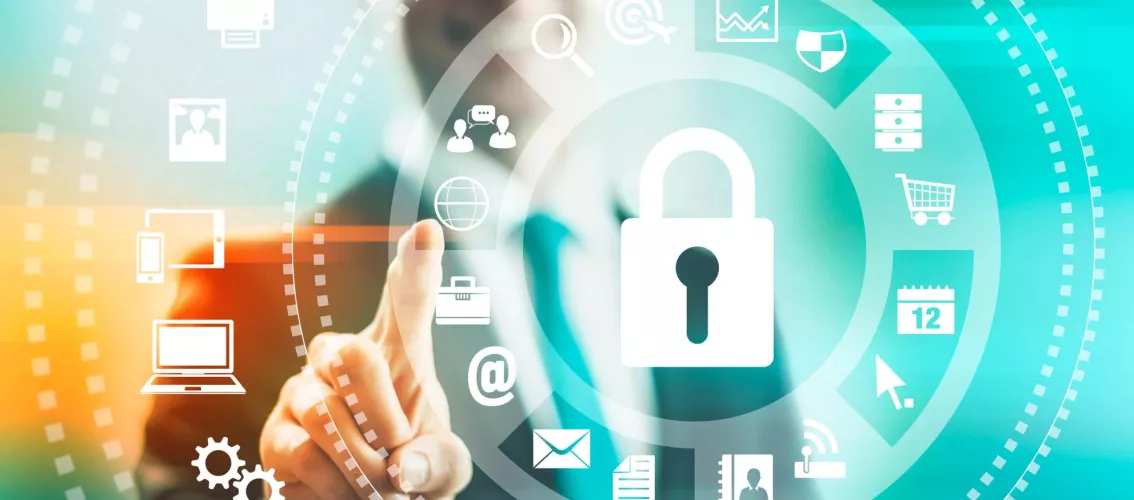
How we use Spring @ConditionalOnProperty Annotation to choose between different security configurations.
Many years ago software applications weren't developed with security in mind. Nowadays security is an integral, important, and necessary part of software development. For this reason, most security protocols and mechanisms have evolved and more and more are introduced each year.
Extend Spring WebSecurityConfigurerAdapter
Spring has lots of built-in standard security mechanisms, but it also provides abstract classes and interfaces with methods which can be overriden and adapted to your own security needs. One of those classes is WebSecurityConfigurerAdapter, which provides a convenient base class for creating a WebSecurityConfigurer "interface in org.springframework.security.config.annotation.web instance.
With this feature you can implement multiple security configurations for an application. In addition, using them in a single module like a jar file, would make them reusable for other applications and save development time for other functionalities. Also, keeping all security configuration, testing, debugging and enhancement in a single module would be much easier.
Choose with @ConditionalOnProperty
There are a variety of security configurations for authentication like Basic, JWT and SAML authentication. The problem that rises almost immediately is how to choose the one we need for each of our applications.
Spring has already introduced some mechanisms to achieve that and we've chosen to use the @ConditionalOnProperty annotation that checks if the specified properties have a specific value. By default the properties of the main application must be present in the Environment like **application.properties** file.
In our security module we have the following java configurations:
**Basic Authentication**
@Configuration
@ConditionalOnProperty(name = "app.security", havingValue = "basic")
public class BasicSecurityConfig extends WebSecurityConfigurerAdapter {
...
}
**JWT Authentication**
@Configuration
@ConditionalOnProperty(name = "app.security", havingValue = "jwt")
public class JwtSecurityConfig extends WebSecurityConfigurerAdapter {
...
}
**SAML Authentication**
@Configuration
@ConditionalOnProperty(name = "app.security", havingValue = "saml")
public class SamlSecurityConfig extends WebSecurityConfigurerAdapter {
...
}
Now let's see how we can achieve different authentication support in new applications.
If for application **A** we want to choose the basic authentication, we just have to add to application.properties the line:
app.security=basic
If for application **B** we want to choose the JWT authentication we just have to add to application.properties the line:
app.security=jwt
Conclusion
In this short post, we've seen the simplicity and flexibility of using the @ConditionalOnProperty annotation and how to use it to load Spring security configurations conditionally.
Take a look at our Web Design and Development and Accessibility evaluations and testing services to see where we can help.