Securing Applications with no code?
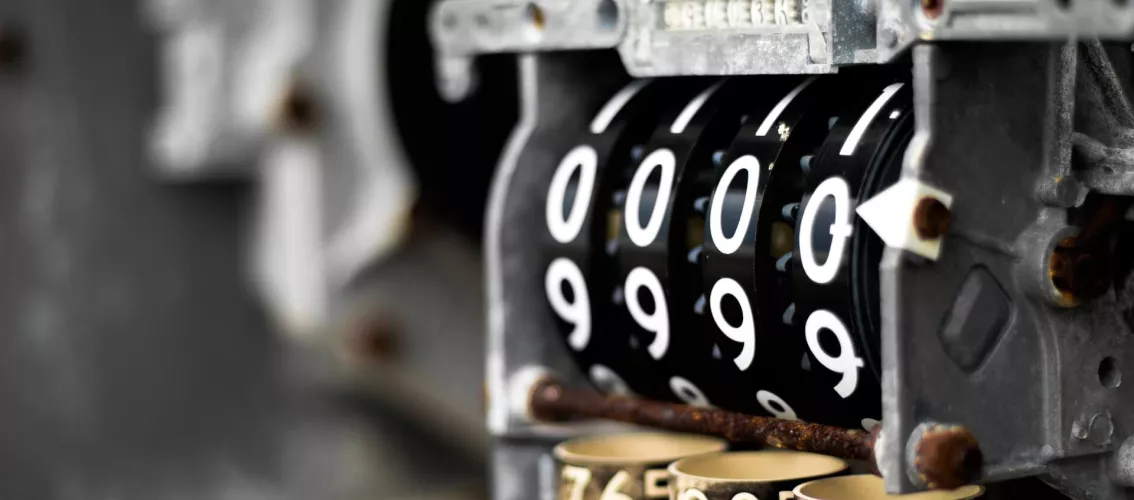
Security has always been a major concern for all applications. In the past, a single security layer was enough to make sure that only the right people could access the application. However, in recent years, modern applications development has been following a “divide and conquer” approach with micro-services architecture dominating the floor. This means that the old monolithic back-end service now breaks into smaller services, each dealing with a specific functionality/responsibility. Thus, an authentication/authorisation service is needed to maintain security for our applications.
As is evident by the use of terms authentication and authorisation, security needs to be provided not only on identity level (by ascertaining that somebody really is who they claim to be), but also on an access rights level (by determining who is allowed to do what).
This is where Keycloak comes to the rescue.
In this blog post, we'll present a simple setup of Keycloak, incorporated into a Spring Boot demo application.
What is Keycloak?
Keycloak offers an Open Source Identity and Access Management solution, which takes over all the fuss of storing/authenticating users and adding authentication and authorisation to our application, since it’s all available out of the box.
As a result, it makes it easier to secure your applications with little to no code, by offering features such as Single-Sign-On (SSO), Identity Brokering and Social Login, an Admin Console, etc. It’s pretty straightforward to download and use, allowing us to almost directly incorporate it into our application.
First step: Authentication
To achieve authentication, we have to set up Keycloak1. Here you'll find a basic setup – including relevant screenshots.
In brief, the steps are the following:
-
Run the standalone.sh script located under <Keycloak_Directory>/bin/standalone.sh (should be standalone.bat for MS Windows)
-
Create a default admin user (since Keycloak doesn't come with a predefined one), by opening http://localhost:8080/auth and filling the form with your preferred credentials.
-
Login to admin console and create a realm (let’s say demo-realm for this example). Realm is the entity that handles a set of users, credentials, roles and groups. Realms are unique and isolated from one another and can only manage users that they control (not users belonging to other realms).
-
Create a user under this realm (for our example, we created a user with username adam).
-
After Clicking Save, additional tabs appear. Navigate to Credentials to initiate a password for adam. After filling the password, please remember (for the purposes of the example) to set Temporary to OFF, to prevent having to update password on first login, and click Set Password.
-
Create a Client (let’s name it api-services for this example), which essentially represents our Spring Boot application. Of course, the Root URL can point to your application’s Root URL.
-
Create Role(s) (optional). This is optional, since the roles are needed only if we want separate users (owning separate roles) to have access to specific services. Let’s assume that – for the purposes of our example – we create a Role named user and assign it to adam.
-
That’s it! A simple setup of creating a Keycloak User is complete! Authentication and access to his realm can now be granted to adam, by hitting a POST to http://localhost:8080/auth/realms/demo-realm/protocol/openid-connect/token , with the necessary header (i.e. Content-Type:application/x-www-form-urlencoded) and Form Parameters:
-
client_id=api-services
-
username=adam
-
password=ad@M123!
-
grant_type=password
The response received shall be a JSON containing the access token to be used by adam when accessing your application’s web services:
{ "access_token": "<access_token>", "expires_in": 300, "refresh_expires_in": 1800, "refresh_token": "<refresh_token>", "token_type": "bearer", "not-before-policy": 0, "session_state": "df52c1a9-abc0-4670-ac76-81aaa5b2118e", "scope": "email profile" }
What about Authorisation?
Authorisation is achieved by incorporating Keycloak configuration into our Spring Boot application. There are several examples existing on the Internet and it would be worthless to reinvent the wheel, especially in a short blog post.
Therefore, we'll use a nice tutorial module that perfectly serves our example, by combining Spring Boot and Keycloak2.
The parts that need to be configured, based on what we described in the previous section are:
-
application.properties
In this file, we'll add our own Keycloak configuration properties, meaning the following,
keycloak.auth-server-url=http://localhost:8080/auth
keycloak.realm=demo-realm
keycloak.resource=api-services
keycloak.public-client=true
keycloak.principal-attribute=preferred_username
In this way, we guarantee that only authenticated users with a valid (not expired) token that belong to the demo-realm have authorised access to the services provided by our Spring Boot application.
-
src/main/java/com/baeldung/keycloak/SecurityConfig.java (optional)
In the void configure method of this class, we can add the role restrictions (only if we created Keycloak Roles in step 7 of the previous section and assigned them to our demo user).
Since the role we created is named user, the configure method contains the .antMatchers(“/customers*”, “/users*”).hasRole(“user”).
If we hadn't previously created a role, then the .hasRole(“user”) wouldn't be needed. On the contrary, if we'd given another role name, it should be .hasRole(“<role_name>”).
With the above modifications, we've a – ready to run – Spring Boot application connected with Keycloak, which is now responsible for the authentication and authorisation of the users. What is left to do is simply add an Authorisation header in our calls to /customers or/and /users, with a value of Bearer <access_token_received_in_previous_section>.
No local database for the application’s users needed, no additional implementation and almost no code at all.
Conclusion
No matter if you specifically choose Keycloak, using Identity Providers with your applications removes a big part of the job from your shoulders. By investing some time to configure the chosen Identity Provider, you save huge amount of time – not to mention the lines of code as well – to implement all the authentication and authorisation mechanisms that will provide security to your application.
So, unless the requirements are that strict, do not re-invent the wheel, go for stable, reliable, existing Identity Providers!
Take a look at our Web Design and Development services to see where we can help.
References
1 https://www.keycloak.org/getting-started/getting-started-zip
2 https://github.com/eugenp/tutorials/tree/master/spring-boot-modules/spring-boot-keycloak